More notes that I took while thinking through today's developments...
I'm going to create a new interface to the UI AND to the class that will allow me to initialise a new dict Protocol object. It will then be available to the user to query while the program is running, and the results will be displayed on the right.
Hmmm
But how is this going to help words?
Within the Words system, whenever there are
1. Undefined words
2. A suitable dictionary available wither locally or remotely
it should try to automatically find definitions for each undefined words.
Fetch from the ManagedObjectContext all instances of the Word entity that have not been defined.
Order them in some way (or not). Importance??
Start from the top of the list and go to the bottom. For each Word object in the array.
[Dictionary define:word]
Place the definition
But I'm getting such varied quantities back from the dictionary. And that's only from the one server, in one database! What about when the possible servers and databases increases!?
The exact results are unpredictable, but they will be within reason. And we know how to handle definition objects well enough. Generally, the first few results fired back from the dictionary are the most relevant.
However, we cannot safely disregard the other definitions.
If the default is inappropriate, then the user must decide which definition they want to be 'Number 1', and promote it to the top of the definitions list. This is the definition that is used for quizzing, word lists etc, however, the alternative definitions are still available.
In the UI, all definitions will be available via an auxiliary window. The main definition will of course be viewable against the original word.
I could probably go right ahead and put the DictProtocolDictionary class right into my existing words prototype, but I want to make sure it is ready and will be able to do everything that I want it to do. But I suppose I can always add to it and modify its behaviour later.
What I think I need to workout next is the UI for this system. Words now has the ability to connect to any server that implements the dict protocol. How is the user going to
1. Create a new dictionary.
2. Specify which dictionary they want to use.
3. Remove Dictionaries.
I also have to make sure there is an internet connection available when the dictionary tries to connect, and to fail gracefully when there isn't (rather than crashing which is what lookup does at the moment!)
Possibly, the user can set any number of dictionaries themselves (or select a suggestion from the list) which then associates itself to the actively selected language.
The special case is that it's not a foreign language to you, in which case you are looking for only definitions, not translations to words. There might be a checkbox to allow the user to specify whether the dictionary is going to be used as translation or a definition dictionary.
I just sketched a preference pane which allows you to choose language, add and remove available dictionaries, check whether they are active or not, a text field to change the server and database, and below that the other dictionary settings.
The application will take from these available dictionaries their definitions.
Therefore dict class should have Cocoa compliant Getters and Setters so I can hook them up to an IB GUI.
Before I set up preferences and the like in Words proper, I need to make sure that the classes can handle all the setters and options etc within the cocoa environment. Can I create more than one dictProtocol dictionary and change it's database etc dynamically. This should be developed in the Lookup application.
Aaand here's the fruits of today's labours. What fun! I can't wait to make it easy for anyone to connect to any dictionary in the world with such a simple interface.
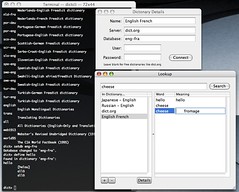
(Click) and look! It's translated cheese to fromage!
Of course, the end goal is to hook the above with what is in this image:
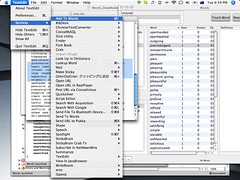
Use the services menu to add you existing word lists (or just a single word) to Words, and have it find the meaning for you, and then save it for later.
fyi
THESE are the dictionaries that reside on dict.org alone!
Database list:
gcide
The Collaborative International Dictionary of English v.0.48
wn
WordNet (r) 2.0
moby-thes
Moby Thesaurus II by Grady Ward, 1.0
elements
Elements database 20001107
vera
Virtual Entity of Relevant Acronyms (Version 1.9, June 2002)
jargon
Jargon File (4.3.1, 29 Jun 2001)
foldoc
The Free On-line Dictionary of Computing (27 SEP 03)
easton
Easton's 1897 Bible Dictionary
hitchcock
Hitchcock's Bible Names Dictionary (late 1800's)
bouvier
Bouvier's Law Dictionary, Revised 6th Ed (1856)
devils
THE DEVIL'S DICTIONARY ((C)1911 Released April 15 1993)
world02
CIA World Factbook 2002
gazetteer
U.S. Gazetteer (1990)
gaz-county
U.S. Gazetteer Counties (2000)
gaz-place
U.S. Gazetteer Places (2000)
gaz-zip
U.S. Gazetteer Zip Code Tabulation Areas (2000)
--exit--
Stop default search here.
afr-deu
Africaan-German Freedict dictionary
afr-eng
Africaan-English Freedict Dictionary
ara-eng
English-Arabic Freedict Dictionary
cro-eng
Croatian-English Freedict Dictionary
cze-eng
Czech-English Freedict dictionary
dan-eng
Danish-English Freedict dictionary
deu-eng
German-English Freedict dictionary
deu-fra
German-French Freedict dictionary
deu-ita
German-Italian Freedict dictionary
deu-nld
German-Nederland Freedict dictionary
deu-por
German-Portugese Freedict dictionary
eng-afr
English-Africaan Freedict Dictionary
eng-ara
English-Arabic FreeDict Dictionary
eng-cro
English-Croatian Freedict Dictionary
eng-cze
English-Czech fdicts/FreeDict Dictionary
eng-deu
English-German Freedict dictionary
eng-fra
English-French Freedict Dictionary
eng-hin
English-Hindi Freedict Dictionary
eng-hun
English-Hungarian Freedict Dictionary
eng-iri
English-Irish Freedict dictionary
eng-ita
English-Italian Freedict dictionary
eng-lat
English-Latin Freedict dictionary
eng-nld
English-Netherlands Freedict dictionary
eng-por
English-Portugese Freedict dictionary
eng-rom
English-Romanian FreeDict dictionary
eng-rus
English-Russian Freedict dictionary
eng-spa
English-Spanish Freedict dictionary
eng-swa
English-Swahili xFried/FreeDict Dictionary
eng-swe
English-Swedish Freedict dictionary
eng-tur
English-Turkish FreeDict Dictionary
eng-wel
English-Welsh Freedict dictionary
fra-deu
French-German Freedict dictionary
fra-eng
French-English Freedict dictionary
fra-nld
French-Nederlands Freedict dictionary
hin-eng
English-Hindi Freedict Dictionary [reverse index]
hun-eng
Hungarian-English FreeDict Dictionary
iri-eng
Irish-English Freedict dictionary
ita-deu
Italian-German Freedict dictionary
jpn-deu
Japanese-German Freedict dictionary
kha-deu
Khasi-German FreeDict Dictionary
lat-deu
Latin-German Freedict dictionary
lat-eng
Latin-English Freedict dictionary
nld-deu
Nederlands-German Freedict dictionary
nld-eng
Nederlands-English Freedict dictionary
nld-fra
Nederlands-French Freedict dictionary
por-deu
Portugese-German Freedict dictionary
por-eng
Portugese-English Freedict dictionary
sco-deu
Scottish-German Freedict dictionary
scr-eng
Serbo-Croat-English Freedict dictionary
slo-eng
Slovenian-English Freedict dictionary
spa-eng
Spanish-English Freedict dictionary
swa-eng
Swahili-English xFried/FreeDict Dictionary
swe-eng
Swedish-English Freedict dictionary
tur-deu
Turkish-German Freedict dictionary
tur-eng
Turkish-English Freedict dictionary
english
English Monolingual Dictionaries
trans
Translating Dictionaries
all
All Dictionaries (English-Only and Translating)
web1913
Webster's Revised Unabridged Dictionary (1913)
world95
The CIA World Factbook (1995)